728x90
반응형
SMALL
임계 처리
임계처리 (thresholding)는 이미지 행렬에서 하나의 픽셀값을 사용자가 지정한 기준값 (threshold)를 사용하여 이진화 (binarization)하는 가장 단순한 필터다. OpenCV에서는 threshold라는 함수로 구현되어 있다. 인수는 다음과 같다.
|
각 임계유형의 결과를 시각화하면 다음과 같다.
import cv2
from skimage.data import coins
img = coins()
maxval = 255
thresh = maxval / 2
_, thresh1 = cv2.threshold(img, thresh, maxval, cv2.THRESH_BINARY)
_, thresh2 = cv2.threshold(img, thresh, maxval, cv2.THRESH_BINARY_INV)
_, thresh3 = cv2.threshold(img, thresh, maxval, cv2.THRESH_TRUNC)
_, thresh4 = cv2.threshold(img, thresh, maxval, cv2.THRESH_TOZERO)
_, thresh5 = cv2.threshold(img, thresh, maxval, cv2.THRESH_TOZERO_INV)
titles = ['원본이미지', 'BINARY', 'BINARY_INV', 'TRUNC', 'TOZERO', 'TOZERO_INV']
images = [img, thresh1, thresh2, thresh3, thresh4, thresh5]
plt.figure(figsize=(9, 5))
for i in range(6):
plt.subplot(2, 3, i+1), plt.imshow(images[i], 'gray')
plt.title(titles[i], fontdict={'fontsize': 10})
plt.axis('off')
plt.tight_layout(pad=0.7)
plt.show()
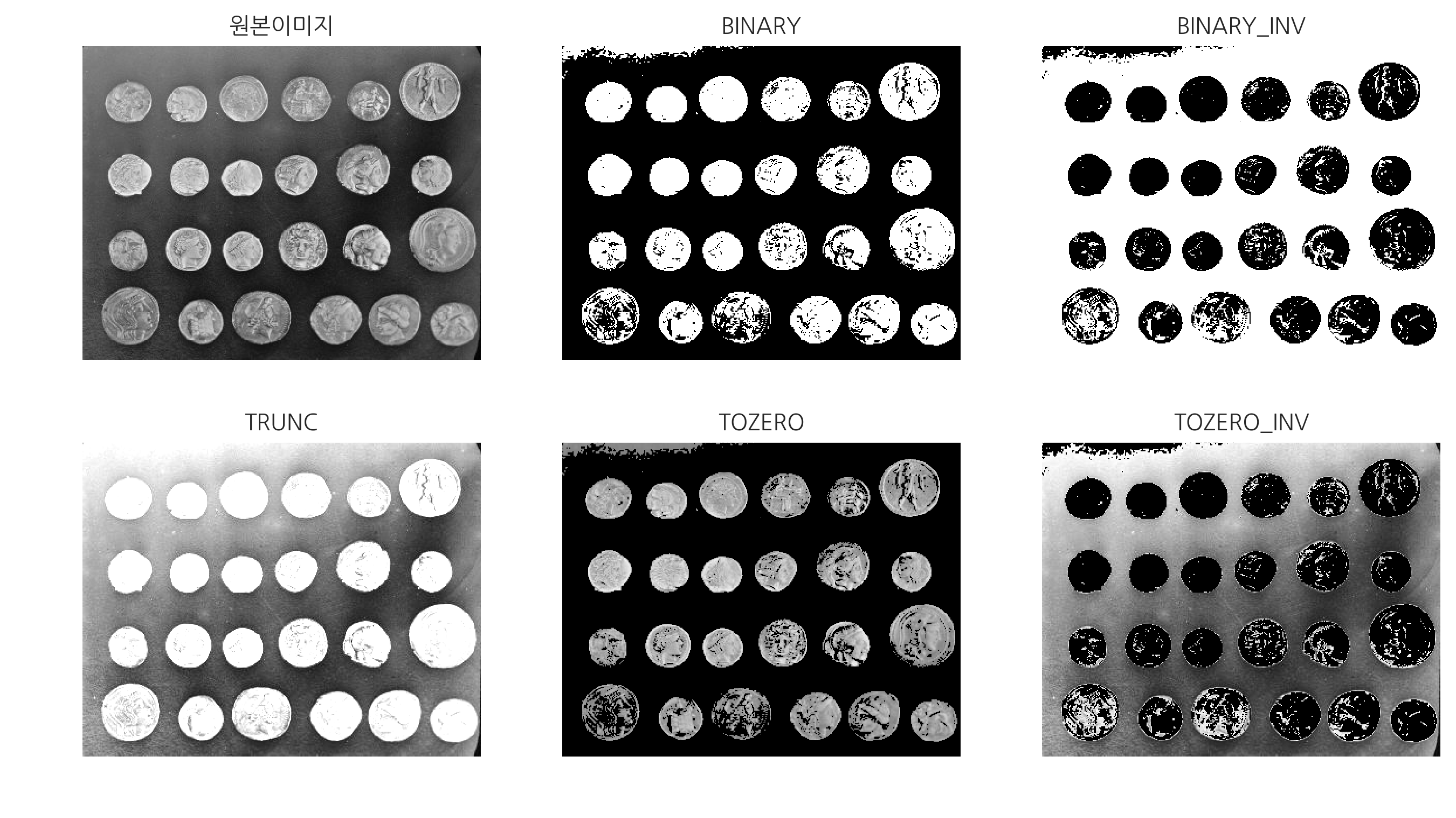
적응임계 처리
임계처리의 경우는 이미지 전체에 하나의 기준값을 적용한다. 적응임계처리는 일정한 영역 내의 이웃한 픽셀들의 값들을 이용하여 해당 영역에 적용할 기준값을 자체적으로 계산한다. OpenCV에서는 adaptiveThreshold 함수로 구현되어 있다.
|
from skimage.data import page
img = page()
maxval = 255
thresh = 126
ret, th1 = cv2.threshold(img, thresh, maxval, cv2.THRESH_BINARY)
k = 15
C = 20
th2 = cv2.adaptiveThreshold(
img, maxval, cv2.ADAPTIVE_THRESH_MEAN_C, cv2.THRESH_BINARY, k, C)
th3 = cv2.adaptiveThreshold(
img, maxval, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, k, C)
images = [img, th1, th2, th3]
titles = ['원본이미지', '임계처리', '평균 적응임계처리', '가우시안블러 적응임계처리']
plt.figure(figsize=(8, 5))
for i in range(4):
plt.subplot(2, 2, i+1)
plt.imshow(images[i], 'gray')
plt.title(titles[i])
plt.axis('off')
plt.tight_layout()
plt.show()
728x90
반응형
LIST
'AI-driven Methodology > CV (Computer Vision)' 카테고리의 다른 글
[Computer Vision] paperswithcode (0) | 2022.08.07 |
---|---|
[Computer Vision] 이미지 변환 (0) | 2022.06.13 |
[Computer Vision] 컨투어 추정 (0) | 2022.06.13 |
[Computer Vision] 이미지 컨투어 (0) | 2022.06.13 |
[Computer Vision] Scikit-Image (0) | 2022.06.13 |